How to Assign Static IP Addresses to Docker Compose Containers
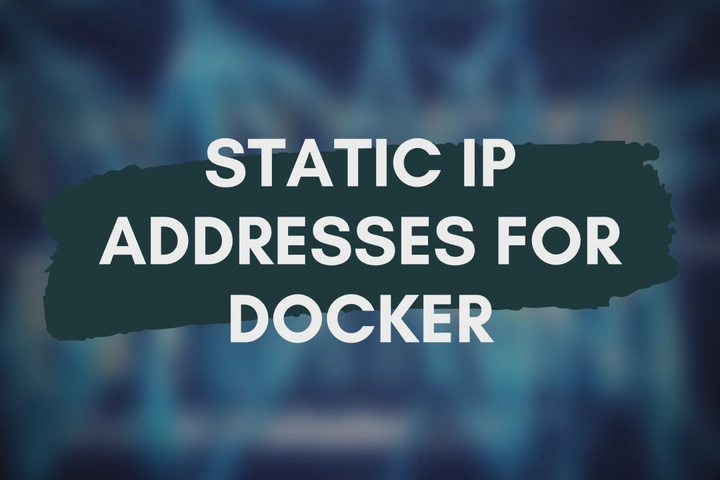
When we start Docker containers with Docker Compose, all the service containers in it will get dynamically assigned IP addresses. For example, if you run the containers in the below docker-compose.yml
file, each of its app service containers can get new or random IP addresses from its default network. This dynamic IP behaviour is not a problem for containers in the same docker-compose.yml
file as they can resolve hostnames out of the box. But the problem is, when you wanted to connect to these containers from the host PC or any other external network, it will be fairly difficult to determine the exact IP address as they do not have any static IP addresses.
version: '3'
services:
app1:
image: 'nginx:1.20.0-alpine'
ports:
- '8181:80'
app2:
image: 'nginx:1.20.0-alpine'
ports:
- '8282:80'
Under that kind of situation, we can assign static IP addresses to Docker Compose service containers so they will always get the exact same IP address. For that, first, we must add a custom network to the docker-compose.yml
file. Make sure to mention an unused subnet or otherwise, you will not be able to start containers.
networks:
appNetwork:
driver: bridge
ipam:
driver: default
config:
- subnet: "172.24.2.0/16"
Then, mention that network (Ex: appNetwork) for each service container that needs a static IP address. Also, make sure to double-check whether the IP address that you are going to assign is in the range of the subnet.
services:
app:
...
networks:
appNetwork:
ipv4_address: 172.24.2.1
Likewise, if you need, you can mention IPv6 addresses with ipv6_address
as well.
Ultimately, the final docker-compose.yml
file should look like something similar to the following.
version: '3'
services:
app1:
image: 'nginx:1.20.0-alpine'
ports:
- '8181:80'
networks:
appnet:
ipv4_address: 172.24.2.1
app2:
image: 'nginx:1.20.0-alpine'
ports:
- '8282:80'
networks:
appnet:
ipv4_address: 172.24.2.2
networks:
appnet:
driver: bridge
ipam:
driver: default
config:
- subnet: "172.24.2.0/16"
Now you can start containers using the docker-compose up
command. Add the -d
flag if you need to start containers in the detached (background) mode.
docker-compose up -d