How to Ignore Python Exceptions with contextlib.suppress()
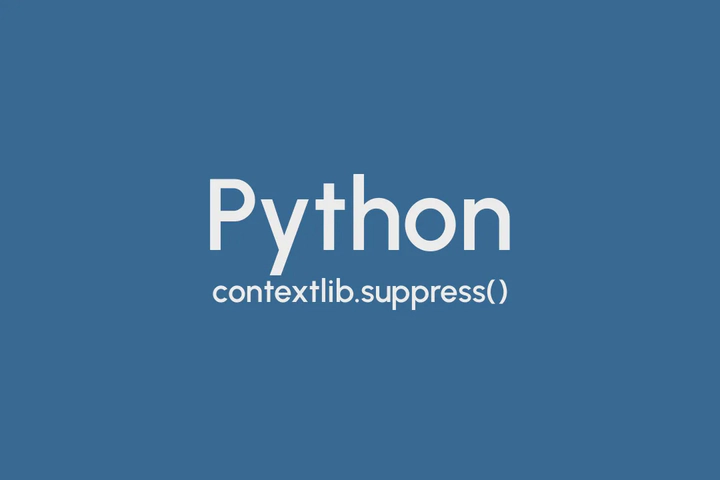
Let’s talk about contextlib.suppress()
, a Python gem that often flies under the radar. Imagine you’re working on a program that needs to delete a file, but you are unsure the related file might not always exist. Under that kind of situation, normally, we write a try-except
block to handle the FileNotFoundError
. Here’s what that looks like:
import os
try:
os.remove("example.txt")
except FileNotFoundError:
pass
This works perfectly as expected, but it feels a little clunky, doesn’t it? All you’re doing is telling Python to ignore a specific exception. Here comes contextlib.suppress()
, the concise and readable way to achieve the same result.
Here’s the same operation using contextlib.suppress()
:
from contextlib import suppress
import os
with suppress(FileNotFoundError):
os.remove("example.txt")
See the difference? The with suppress(FileNotFoundError):
line wraps the operation you want to perform. If a FileNotFoundError
occurs, Python quietly suppresses it and continues executing the rest of the code. No extra lines, just clean and easily readable code.
The suppress()
function comes from the contextlib
module, which is packed with tools for managing resources and contexts. It takes one or more exception types as arguments and works as a context manager. Any exceptions of the specified types raised within the with
block are automatically caught and ignored.
This makes it perfect for scenarios where you want to skip over certain errors without interrupting the flow of your program. For instance, suppose you’re cleaning up temporary files in a directory:
import os
from contextlib import suppress
files_to_delete = ["temp1.txt", "temp2.txt", "temp3.txt"]
for file in files_to_delete:
with suppress(FileNotFoundError):
os.remove(file)
Now, even if one or more of the files doesn’t exist, the loop will not break. Instead, Python will suppress the FileNotFoundError
for the missing files and continue to the next one.
Alright, let’s discuss a common question. Doesn’t suppressing exceptions make debugging more harder? Actually, it depends according to your requirements and on how you are going to use it. The suppress()
is the good for situations like you clearly know they are just safe to ignore. If there is any uncertainty about the exception’s impact, a traditional try-except
block will be more suitable as it always allows for logging and more control.
Furthermore, suppose you are developing a tool that processes multiple input files. Some of these files might be missing, but that’s okay, you just want to process the ones that exist. Here’s how you could use suppress()
for that.
from contextlib import suppress
file_paths = ["data1.csv", "data2.csv", "data3.csv"]
for path in file_paths:
with suppress(FileNotFoundError):
with open(path, "r") as file:
print(f"Processing {path}")
# Add file processing logic here
Using suppress()
here keeps the code clean and readable. Any missing files are simply skipped without adding extra noise to the logic.
Now let’s talk performance. The beauty of suppress()
is that it has negligible overhead—it’s essentially syntactic sugar around a try-except
block. However, its impact on readability and maintainability is huge. Cleaner code is easier to debug, extend, and hand off to teammates.
The contextlib.suppress()
is a fantastic tool when you just need to ignore specific and predictable exceptions. Give it a try in your next project!